I have a PHP application I started writing back in 2010 and worked on it until 2016. Years later, I want to containerize the application since that is what we do. Also, I want to revisit the application and make some improvements. When I first started the project, the development landscape was a lot different back then which also includes tooling.
Current Production State
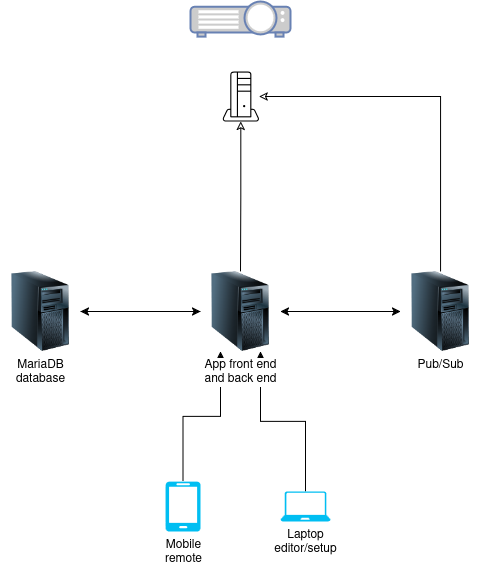
Presently the app is running in production. There is a server that serves the front end and back end running Apache httpd and PHP is being handled by PHP-FPM. There is another server that is running Crossbar to facilitate the pub/sub via WebSockets. Finally, there is a MariaDB database server. In the beginning, the database server was MySQL.
Containerization
With the above architecture in mind, we set out on the path of containerization.
MariaDB
The easiest service to containerize was the MariaDB database. The below Docker compose service gives us a MariaDB database server.
db:
image: mariadb:10.11
environment:
- MARIADB_ROOT_PASSWORD=root-password
- MARIADB_USER=worshipaid
- MARIADB_PASSWORD=password
- MARIADB_DATABASE=worshipaid
ports:
- 127.0.1.1:3306:3306
volumes:
- ./db:/var/lib/mysql
restart: unless-stopped
At this point we restore a dump of production into the containerized database. It was that easy.
Crossbar
Let us start with the Docker compose service block:
wamp:
image: crossbario/crossbar:pypy3-20.12.1
ports:
- 8081:8080
volumes:
- ./crossbar/config.json:/node/.crossbar/config.json
restart: unless-stopped
This service needs to be reachable by both the application server and the mobile remote. We expose this service on port 8081
since 8080
will be taken by the web server later on. Configuration of this service is done with a config.json
file, so we took a copy of production, removed the TLS config and bind mount it into the container where Crossbar is expecting to find the config.
Web Server and PHP-FPM
In our production setup, Apache httpd and PHP-FPM live on the same server. We could have attempted to create a container image that does the same thing, but I took this opportunity to set things up as two separate services because I was feeling adventurous and wanted to run the application via a separate PHP-FPM container.
backend:
# image: php:7.2-fpm
build:
context: ../
dockerfile: docker/Dockerfile
environment:
- WORSHIPAID_ADMIN_PASSWORD=adminpw
- WORSHIPAID_USER_PASSWORD=userpw
- MARIADB_HOST=db
- MARIADB_USER=worshipaid
- MARIADB_PASSWORD=password
- MARIADB_DATABASE=worshipaid
- WAMP_HOST=wamp
- WAMP_PORT=8080
- WAMP_TLS=false
- WAMP_FRONTEND_HOST=localhost
- WAMP_FRONTEND_PORT=8081
- WAMP_FRONTEND_TLS=false
user: 1000:1000
volumes:
- ../:/var/www/html
frontend:
image: httpd:2.4
ports:
- 8080:80
volumes:
- ./httpd.conf:/usr/local/apache2/conf/httpd.conf
- ../:/opt/worshipaid
frontend
Starting with the frontend service, it is Apache httpd. We had to make a few modifications to httpd.conf
such as adjusting the DocumentRoot
and its <Directory>
config. We also added the following to pass any PHP requests to the backend service
ProxyPassMatch ^/(.*\.php(/.*)?)$ fcgi://backend:9000/var/www/html/$1
We also needed to enable the appropriate modules: mod_proxy
, mod_proxy_fcgi
.
The modified config is injected into the container through a bind mount and our application is mounted into our DocumentRoot
.
backend
This one was an interesting exercise. In production, the front end and back end live on the same server, but now we are putting them in different containers.
In the Docker compose service, there is a build
config that references a Dockerfile
.
FROM php:7.4-fpm
COPY ./composer.json ./composer.lock /var/lib/worshipaid/
RUN apt update && \
apt install -y --no-install-recommends git unzip && \
docker-php-ext-install pdo_mysql && \
cd /var/lib/worshipaid && \
curl -sS https://getcomposer.org/installer | php && \
php composer.phar install
ENV COMPOSER_DIR=/var/lib/worshipaid
We copy composer.json
and composer.lock
into a directory that in theory should not get masked when we bind mount our application into the container. For our use case, that place is going to be /var/lib/worshipaid
.
Composer requires git and unzip to do its dependency management things, so we install that via apt
.
For database functionality, we need to have MySQL extension enabled. The image has a helper script called docker-php-ext-install
that takes care of that for us.
Lastly, we navigate to /var/lib/worshipaid
and install our dependencies and also set an environment variable such that we can direct our application to look for the framework in that location instead of where it might usually be.
In the Docker compose service config, there are a bunch of environment variables. We will cover them soon.
Supporting Environment Variables
Now that we are containerizing the application, we want to minimize the differences from image to image due to things such as configuration. One approach to this is to make things configurable through environment variables.
The low-hanging fruit are things such as database connections. In our application’s configuration file, we replace hardcoded values with variables such as $_ENV['MARIADB_HOST']
.
Sometimes it is also necessary to introduce additional configuration parameters to support a new environment.
With regards to Crossbar, that service is being accessed both internally and externally. Originally there was one set of parameters we needed to set, now there are two sets: one for the back end to communicate with internally, one for the external clients to connect to. As a result, we had to write some code to support the additional parameters and make them configurable via front end-specific environment variables.
For the backend image, we set a COMPOSER_DIR
environment variable inside of the image. To make use of this, we updated our entry point to load the framework from COMPOSER_DIR
instead of looking in the old location which is the current folder.
Wrapping Up
Overall, containerizing this application was not too bad. The potentially challenging bits were the Crossbar pub/sub service and adjusting things such that the application operates with separate front end and a back end containers. Although the containerization exercise sets us up for a development environment, the effort to build live/container image(s) should be pretty trivial. Below is what the architecture would look like now that we are containerized.
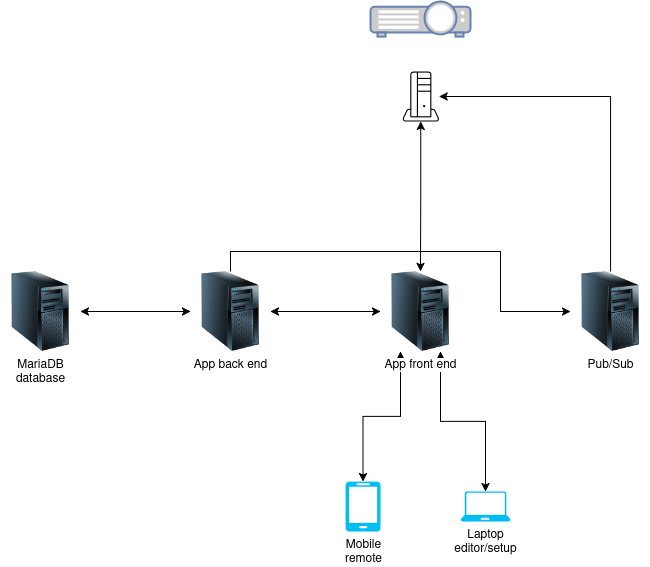
As for what WorshipAid is, that is coming soon ™